python基础基础初识2
发布于 2021-04-17 05:48 ,所属分类:知识学习综合资讯
目录
流程控制语句while
格式化输出
基本运算符
编码
详解
流程控制语句while
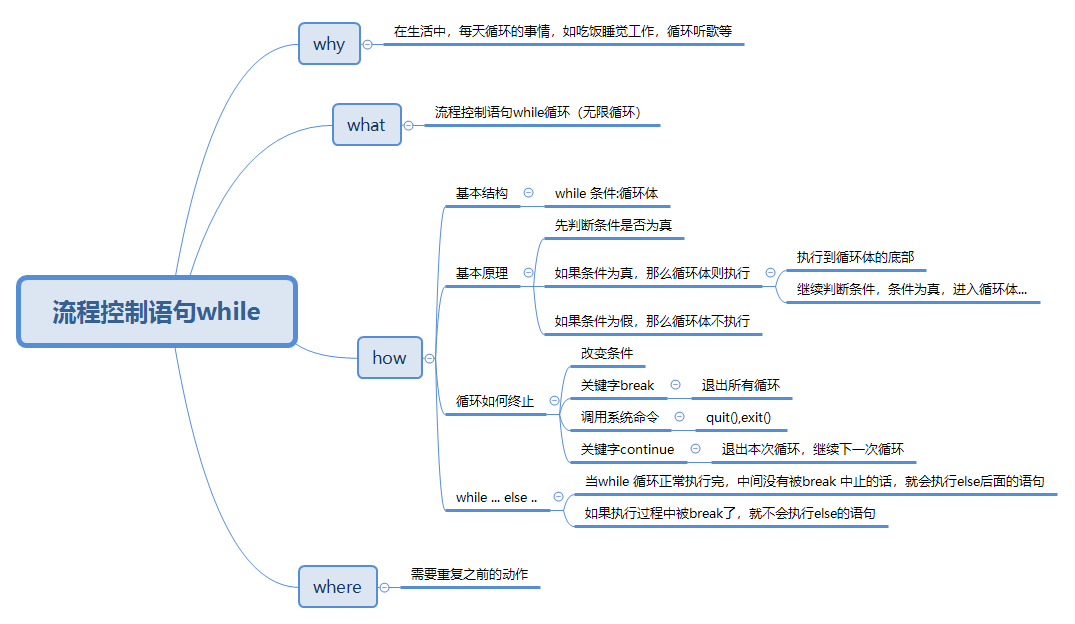
(点击查看大图)
基本结构
while 条件:
循环体
# 如果条件为真,那么循环体则执行
# 如果条件为假,那么循环体不执行
(左右滑动查看完整代码)
循环如何终止
终止循环的第一个方法:改变条件
'''1.改变条件'''
# 求1-100所有数的和.
count = 1
sum = 0
while count < 101:
sum += count
count += 1
print(sum) # 输出为:5050
(左右滑动查看完整代码)
终止循环的第二个方法:break
break的用法:循环中,只要遇到break马上退出循环。
'''2.关键字break'''
# 打印1-100所有的偶数
count = 1
while True:
if count % 2 == 0:
print(count)
count = count + 1
if count == 101:
break
(左右滑动查看完整代码)
终止循环的第三个方法:continue
continue 用于终止本次循环,继续下一次循环。
'''3.关键字continue'''
# 打印 1 2 3 4 5 6 8 9 10
count = 0
while count < 10:
count = count + 1
if count == 7:
continue
print(count)
(左右滑动查看完整代码)
while ... else ...
while 后面的else 作用是指:当while 循环正常执行完,中间没有被break 中止的话,就会执行else后面的语句。如果被break打断,则不执行else语句。
count = 1
while count < 5:
print(count)
if count == 2:
break
count = count + 1
else:
print('循环正常执行完了')
'''
输出为:
1
2
'''
(左右滑动查看完整代码)
格式化输出
格式化输出的作用是:让字符串的某些位置变成动态可变的、可传入的。
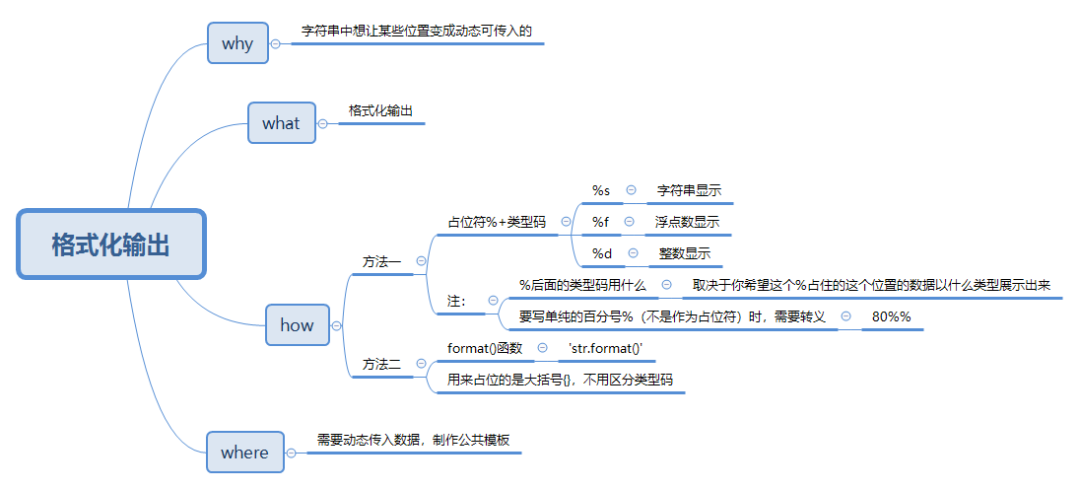
(点击查看大图)
方法一
格式符+类型码 | 含义 |
%s | 字符串显示 |
%f | 浮点数显示 |
%d | 整数显示 |
%后面的类型码用什么,取决于你希望这个%占住的这个位置的数据以什么类型展示出来,如果你希望它以字符串形式展示,那就写%s,如果你希望它以整数形式展示,那就写%d。
name = 'pamela'
age = 18
msg = '%s, %d' % (name, age)
print(msg) # 输出为:pamela, 18
(左右滑动查看完整代码)
方法二
format()函数是从 Python2.6 起新增的一种格式化字符串的函数,功能比方法一更强大。
format()函数用来占位的是大括号{},不用区分类型码。
具体的语法是:'str.format()',而不是方法一提到的'str % ()'。
而且,它对后面数据的引用更灵活,不限次数,也可指定对应关系。
name = 'pamela'
age = 18
msg = '{}, {}'.format(name, age)
print(msg) # 输出为:pamela, 18
# 指定对应关系
msg1 = '{1}, {0}, {1}'.format(name, age)
print(msg1) # 输出为:18, pamela, 18
msg2 = '{name}, {age}'.format(age=age, name=name)
print(msg2) # 输出为:pamela, 18
(左右滑动查看完整代码)
基本运算符
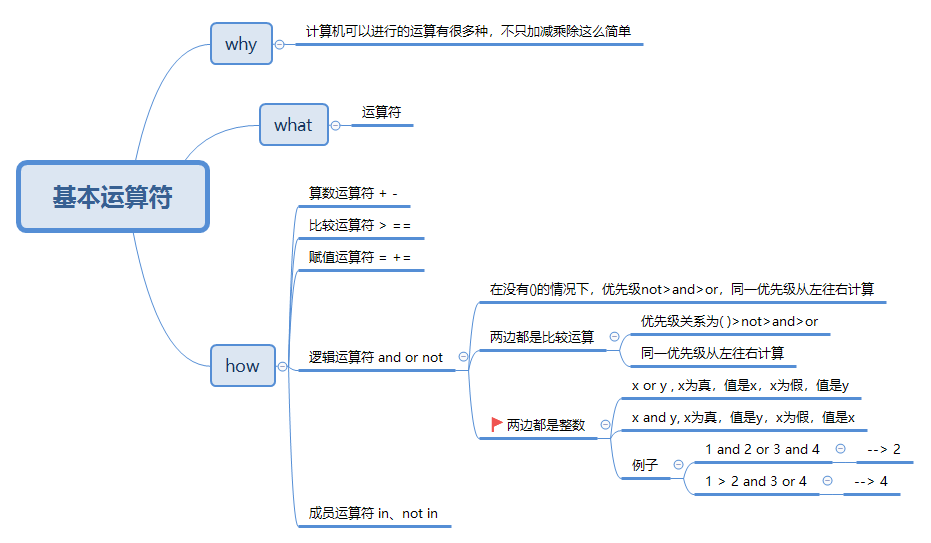
(点击查看大图)
算数运算
以下假设变量:a=10,b=20
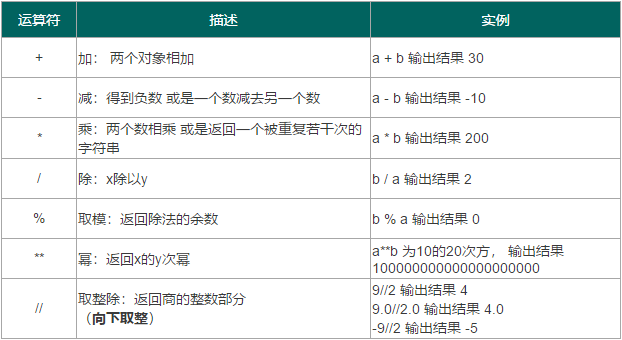
(点击查看大图)
比较运算
以下假设变量:a=10,b=20
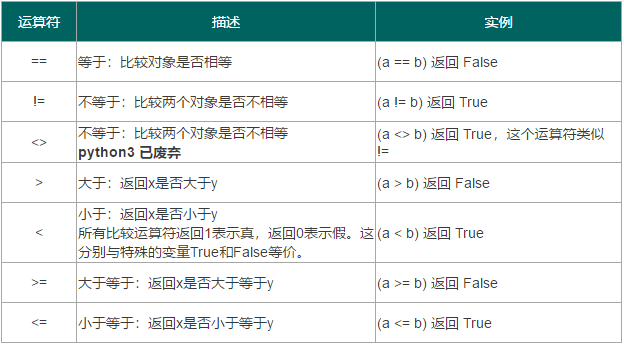
(点击查看大图)
赋值运算
以下假设变量:a=10,b=20
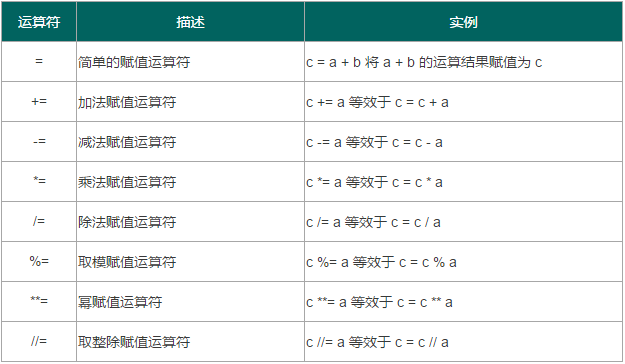
(点击查看大图)
逻辑运算
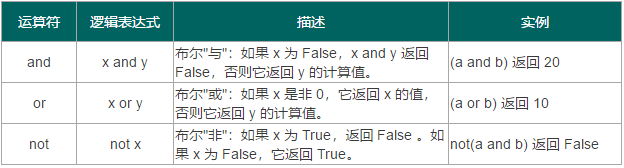
(点击查看大图)
优先级关系为( )>not>and>or,同一优先级从左往右计算
两边都是比较运算:
print(3 > 4 or 4 < 3 and 1 == 1) # 输出为:False
print(1 < 2 and 3 < 4 or 1 > 2) # 输出为:True
print(2 > 1 and 3 < 4 or 4 > 5 and 2 < 1) # 输出为:True
print(1 > 2 and 3 < 4 or 4 > 5 and 2 > 1 or 9 < 8) # 输出为:False
print(1 > 1 and 3 < 4 or 4 > 5 and 2 > 1 and 9 > 8 or 7 < 6) # 输出为:False
print(not 2 > 1 and 3 < 4 or 4 > 5 and 2 > 1 and 9 > 8 or 7 < 6) # 输出为:False
(左右滑动查看完整代码)
两边都是整数:
x or y,x为真,值是x;x为假,值是y。
x and y,x为真,值是y;x为假,值是x。
print(8 or 4) # 输出为:8
print(0 and 3) # 输出为:0
print(0 or 4 and 3 or 7 or 9 and 6) # 输出为:3
(左右滑动查看完整代码)
成员运算
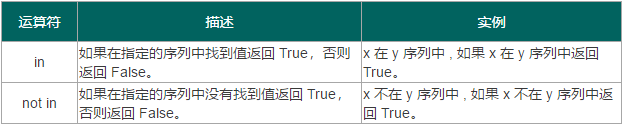
(点击查看大图)
判断子元素是否在原字符串(字典,列表,元祖,集合)中:
print('喜欢' in 'dkdklf喜欢hfas') # 输出为:True
print('a' in 'bcde') # 输出为:False
print('b' not in 'qwertyu') # 输出为:True
(左右滑动查看完整代码)
Python运算符优先级
从最高到最低优先级的所有运算符:
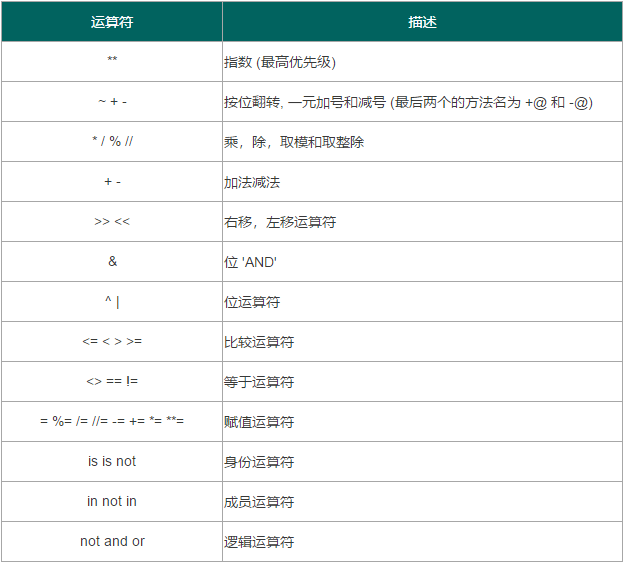
(点击查看大图)
编码
编码的本质就是让只认识0和1的计算机,能够理解我们人类使用的语言符号,并且将数据转换为二进制进行存储和传输。
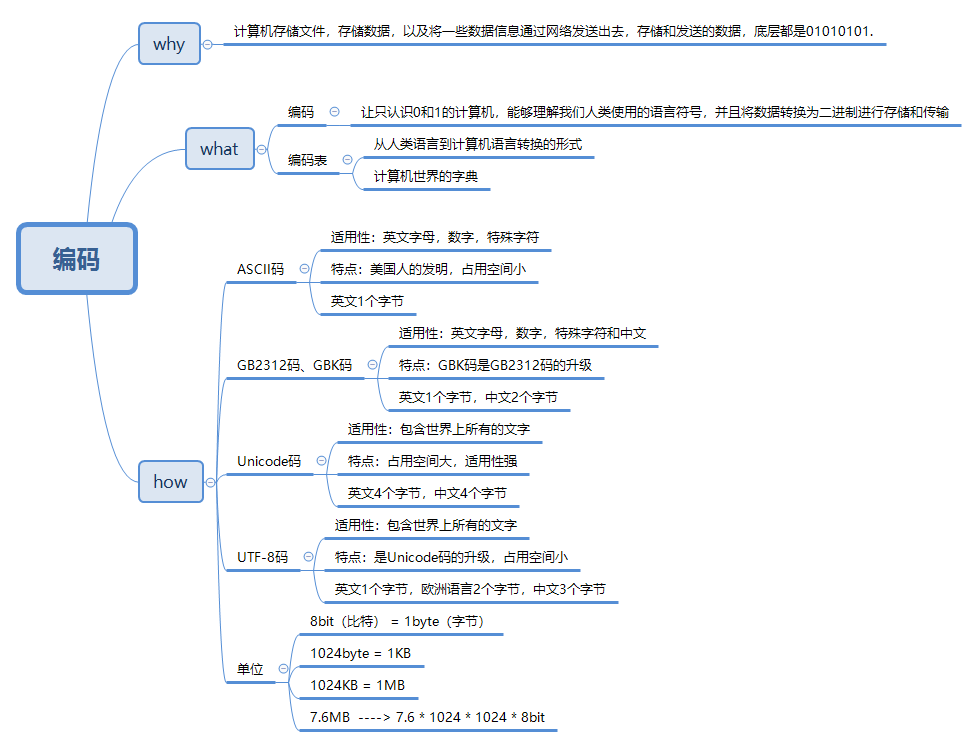
(点击查看大图)
相关资源